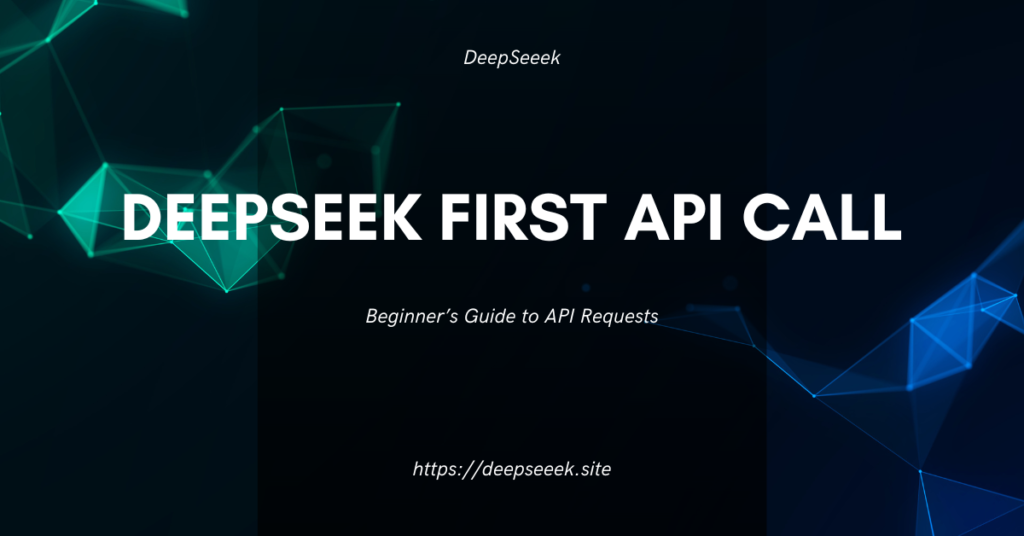
Deepseek First API Call
In the modern tech landscape, APIs (Application Programming Interfaces) serve as the backbone of software development, enabling applications to communicate with each other seamlessly. Whether you’re a beginner exploring API integrations or a developer making your Deepseek first API call, understanding the process is crucial for smooth functionality.
This beginner-friendly guide will walk you through everything you need to know about making your Deepseek first API call, from setup to troubleshooting. By the end of this tutorial, you’ll confidently send API requests, retrieve responses, and integrate APIs into your projects.
What is an API and Why is It Important?
An API (Application Programming Interface) is a set of rules and protocols that allows different software applications to communicate. Instead of building everything from scratch, APIs let developers access pre-built functionalities and data from external services.
🔹 Why Are APIs Important?
- Simplifies Development – Saves time by allowing integration with external services.
- Increases Efficiency – Enables applications to share data without manual intervention.
- Enhances User Experience – Allows real-time updates, like weather data, social media feeds, and payment processing.
- Scalability – Helps applications grow by integrating new functionalities via API requests.
When making your Deepseek first API call, you’ll be tapping into a system that provides structured data without building complex infrastructure from scratch.
Deepseek First API Call | Beginner’s Guide to API Requests
1. Understanding API Requests and Responses
Before making your Deepseek first API call, it’s essential to understand how API requests and responses work.
🔹 API Request Structure:
When interacting with an API, you send a request to an API endpoint using an HTTP method. The most commonly used methods are:
✔ GET – Retrieves data from the API.
✔ POST – Sends new data to the API.
✔ PUT – Updates existing data in the API.
✔ DELETE – Removes data from the API.
A typical API request consists of:
- Endpoint URL – The web address where the request is sent.
- Headers – Metadata such as authentication tokens.
- Body – Data payload (used in POST/PUT requests).
🔹 API Response Structure:
Once the request is processed, the API returns a response in a structured format, typically JSON.
Example JSON response:
jsonCopyEdit{
"status": "success",
"data": {
"message": "API call successful"
}
}
Each response includes:
✔ Status Code – Indicates if the request was successful (e.g., 200 for success, 404 for not found).
✔ Response Body – Contains the requested data or error message.
2. Setting Up for Your Deepseek First API Call
Before making your Deepseek first API call, you need to complete the following setup steps:
Step 1: Obtain API Credentials
Many APIs, including Deepseek, require authentication to prevent unauthorized access. Check if you need:
✔ API Key – A unique identifier for secure access.
✔ OAuth Token – A more secure authentication method for user-based access.
🔹 How to Obtain an API Key for Deepseek:
1️⃣ Sign up on the Deepseek website and create an account.
2️⃣ Navigate to the Developer Section and generate an API Key.
3️⃣ Save your API Key securely (do not share it publicly).
Step 2: Install Required Tools
To interact with the API, you can use:
✔ Postman – A GUI-based API testing tool.
✔ cURL – A command-line tool for sending API requests.
✔ Python (Requests Library) – Ideal for scripting API interactions.
3. Making Your First API Call to Deepseek
Now that everything is set up, let’s make a Deepseek first API call step by step.
Method 1: Using cURL (Command Line)
If you prefer the command line, you can use cURL to make a GET request.
shCopyEditcurl -X GET "https://api.deepseek.com/v1/data" -H "Authorization: Bearer YOUR_API_KEY"
This command:
✔ Sends a GET request to Deepseek API.
✔ Includes an authentication token in the request header.
Method 2: Using Postman
1️⃣ Open Postman and create a new request.
2️⃣ Enter the API URL (e.g., https://api.deepseek.com/v1/data
).
3️⃣ Add Authorization Header (Bearer YOUR_API_KEY
).
4️⃣ Click “Send” and check the response.
Method 3: Using Python (Requests Library)
For developers using Python, the requests
library makes API interactions simple.
pythonCopyEditimport requests
url = "https://api.deepseek.com/v1/data"
headers = {"Authorization": "Bearer YOUR_API_KEY"}
response = requests.get(url, headers=headers)
print(response.json())
✔ This script sends a request and prints the JSON response.
4. Troubleshooting Common API Errors
When making your Deepseek first API call, you may encounter errors. Here’s how to fix common issues:
Error Code | Issue | Solution |
---|---|---|
400 Bad Request | Incorrect API parameters | Check request body and parameters |
401 Unauthorized | Invalid API Key | Ensure API Key is correct and active |
403 Forbidden | Insufficient permissions | Verify API access settings |
404 Not Found | Invalid endpoint | Check URL spelling and endpoint |
500 Internal Server Error | API service issue | Retry after some time or contact support |
If you get an error, always check:
✔ The API documentation for the correct request format.
✔ Your authentication credentials to ensure they’re valid.
✔ The Deepseek API status page for potential downtime.
FAQs About Deepseek First API Call
1. What is the Deepseek API used for?
The Deepseek API allows developers to retrieve data, analytics, and AI-driven insights for various applications. It is commonly used in machine learning, data processing, and automation.
2. Do I need an API key to make my Deepseek first API call?
Yes. Most Deepseek API endpoints require authentication using an API Key or OAuth token. You can obtain this by registering on the Deepseek developer portal.
3. Can I test the API before integrating it?
Yes! You can use Postman or cURL to send test requests before integrating the API into your application.
4. What programming languages can I use to interact with the Deepseek API?
You can use any programming language that supports HTTP requests, such as:
✔ Python (Requests library)
✔ JavaScript (Fetch API, Axios)
✔ Node.js (Axios, Express)
✔ Java (OkHttp, Apache HttpClient)
5. How do I handle rate limits on the Deepseek API?
When working with the Deepseek API, you may encounter rate limits that restrict the number of requests you can make within a specific time frame. If you exceed the limit, the API will return a 429 Too Many Requests
error, temporarily blocking further requests.
To ensure smooth integration and efficient use of API resources, follow these best practices to manage and avoid rate limits effectively:
Understanding API Rate Limits
API rate limits are implemented to:
✔ Prevent excessive server load and ensure fair usage.
✔ Improve API performance by distributing requests evenly.
✔ Avoid service disruptions caused by high-traffic applications.
Each API provider, including Deepseek, has specific rate limit rules, which may vary depending on:
- User subscription plans (Free, Premium, Enterprise).
- Type of API request (data retrieval, model execution, etc.).
- Time intervals (per second, per minute, per hour, etc.).
To check your current rate limits, review the Deepseek API documentation or inspect the API response headers.
How to Avoid 429 Too Many Requests Errors
1. Optimize API Request Handling
Efficient request management ensures you stay within the API’s rate limits while maintaining performance.
🔹 Best Practices for Request Optimization:
✔ Avoid unnecessary API calls – Only request data when needed.
✔ Batch requests – Instead of multiple small requests, group them into a single request when possible.
✔ Use efficient pagination – If fetching large datasets, retrieve data in chunks rather than making multiple calls.
🔹 Example: Instead of making 10 separate API calls for different user profiles, fetch all profiles in one batch request if supported by Deepseek API.
2. Implement Caching to Reduce Redundant Requests
Caching allows you to store API responses temporarily, reducing the need for repeated requests for the same data.
🔹 How Caching Helps with Rate Limits:
✔ Reduces API calls by storing frequently used data.
✔ Improves response time by serving cached data instead of making a new API request.
✔ Minimizes the risk of exceeding the rate limit.
🔹 Implementation Methods:
- Use server-side caching (Redis, Memcached) for storing API responses.
- Implement browser caching if working with client-side applications.
- Set expiration times to refresh cached data periodically.
🔹 Example: If fetching stock prices from Deepseek API, store the latest data for 5 minutes instead of making a new request every second.
3. Respect the Rate Limits & Retry Strategy
When you approach the API rate limit, it’s important to slow down your requests or implement a retry mechanism.
🔹 Steps to Handle Rate Limits Effectively:
✔ Monitor API response headers – Many APIs return a Retry-After
header, indicating when to send the next request.
✔ Implement exponential backoff – A retry strategy where failed requests are retried with increasing time intervals.
✔ Use queueing mechanisms – If multiple requests need to be processed, implement a queue system to control request frequency.
🔹 Example of Exponential Backoff (Python):
pythonCopyEditimport time
import requests
def api_request_with_backoff(url, headers, max_retries=5):
retry_delay = 1 # Initial delay in seconds
for attempt in range(max_retries):
response = requests.get(url, headers=headers)
if response.status_code == 429: # Too Many Requests
print(f"Rate limit exceeded. Retrying in {retry_delay} seconds...")
time.sleep(retry_delay)
retry_delay *= 2 # Exponential increase
else:
return response.json()
print("Max retries reached. Request failed.")
return None
✔ This function detects a 429 error, waits before retrying, and gradually increases the delay if the error persists.
4. Upgrade to Higher Rate Limit Plans (If Necessary)
If your application frequently exceeds the rate limits, consider upgrading to a higher-tier Deepseek API plan that offers increased request limits.
🔹 How to Check & Upgrade Your Plan:
✔ Visit the Deepseek API dashboard to check your current usage and limits.
✔ If exceeding limits regularly, explore premium or enterprise plans.
✔ Contact Deepseek support for custom API solutions if your application requires high-volume requests.
🔹 Example: A business application that makes thousands of real-time API requests daily may need to switch from a free plan (limited requests) to a premium subscription with higher limits.
Final Thoughts: Efficient API Usage for Better Performance
Handling API rate limits effectively ensures smooth application performance and prevents disruptions when working with the Deepseek API.
💡 Key Takeaways:
✔ Monitor API requests and optimize efficiency.
✔ Implement caching to reduce redundant requests.
✔ Use retry strategies when rate limits are hit.
✔ Upgrade to a higher-tier API plan if necessary.
By following these best practices, you can avoid 429 errors, enhance application performance, and maximize API efficiency while working with Deepseek first API call requests. 🚀
Final Thoughts: Mastering Your Deepseek First API Call
Making your Deepseek first API call is the first step toward API integration. With the right setup, authentication, and troubleshooting knowledge, you can efficiently retrieve data and automate tasks using Deepseek’s API.
Read more: